Principios SOLID en Desarrollo de Software
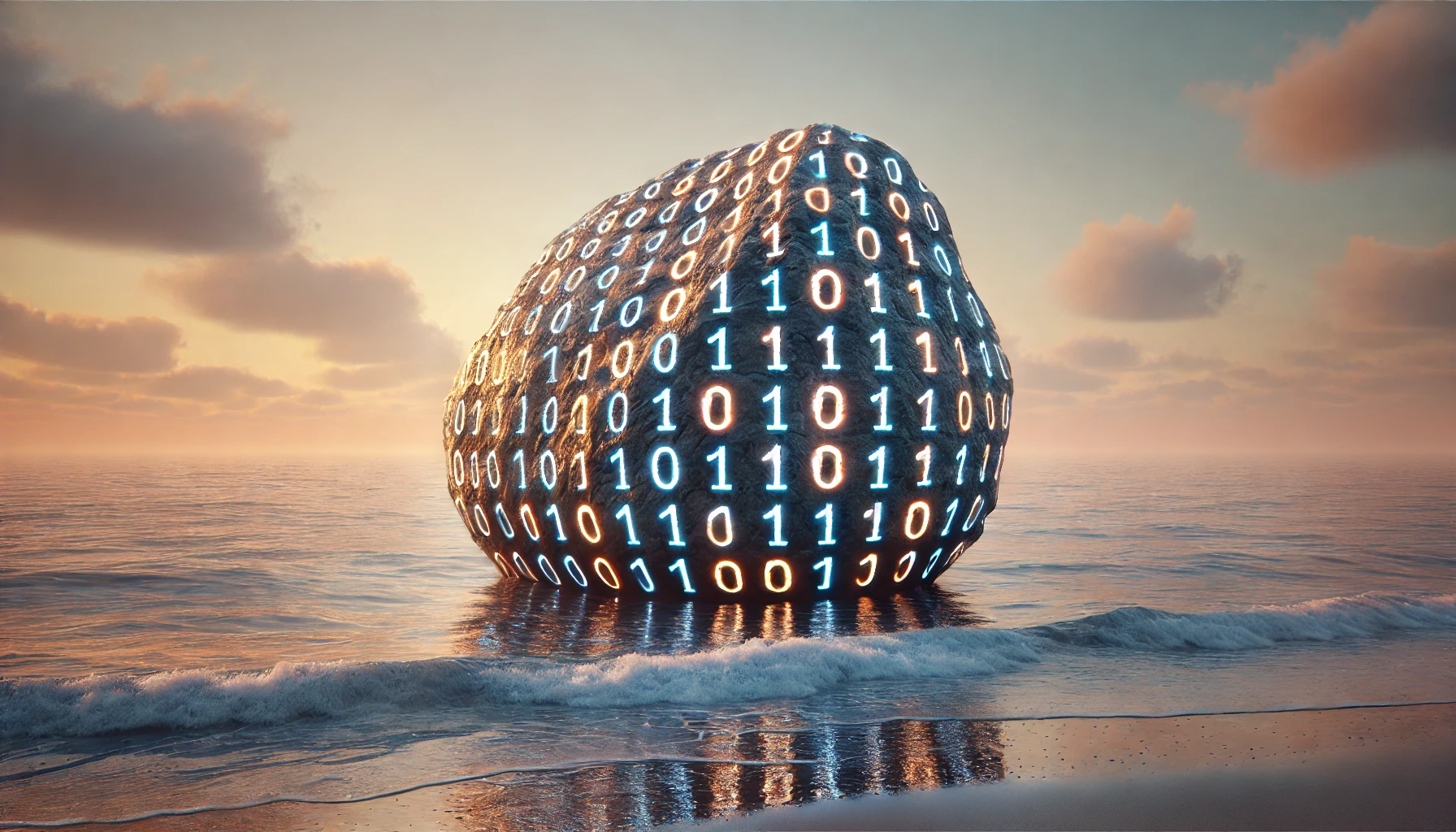
Blog: Mark Harmsen - 07 de febrero 152 vistas
SOLID is an acronym representing five key principles of object-oriented programming (OOP) and software design. These principles help developers create software that is more maintainable, scalable, and robust.
1. Single Responsibility Principle (SRP)
A class should have only one reason to change. Each class should have only one responsibility.
class ReportGenerator {
void generateReport() {
// Logic for generating a report
}
}
class ReportPrinter {
void printReport() {
// Logic for printing a report
}
}
2. Open/Closed Principle (OCP)
Software entities should be open for extension, but closed for modification.
interface PaymentMethod {
void pay(double amount);
}
class CreditCardPayment implements PaymentMethod {
public void pay(double amount) {
// Credit card payment logic
}
}
class PayPalPayment implements PaymentMethod {
public void pay(double amount) {
// PayPal payment logic
}
}
3. Liskov Substitution Principle (LSP)
Subtypes must be substitutable for their base types without altering correctness.
abstract class Bird {}
interface CanFly {
void fly();
}
class Sparrow extends Bird implements CanFly {
public void fly() {
// Sparrow can fly
}
}
class Penguin extends Bird {
// No fly method, avoiding LSP violation
}
4. Interface Segregation Principle (ISP)
A class should not be forced to implement interfaces it does not use.
interface Workable {
void work();
}
interface Eatable {
void eat();
}
class Human implements Workable, Eatable {
public void work() {}
public void eat() {}
}
class Robot implements Workable {
public void work() {}
}
5. Dependency Inversion Principle (DIP)
High-level modules should not depend on low-level modules. Both should depend on abstractions.
interface Keyboard {}
interface Monitor {}
class MechanicalKeyboard implements Keyboard {}
class LEDMonitor implements Monitor {}
class Computer {
private Keyboard keyboard;
private Monitor monitor;
public Computer(Keyboard keyboard, Monitor monitor) {
this.keyboard = keyboard;
this.monitor = monitor;
}
}
Keyboard keyboard = new MechanicalKeyboard();
Monitor monitor = new LEDMonitor();
Computer computer = new Computer(keyboard, monitor);
Benefits of SOLID Principles
- Maintainability – Code is easier to understand and modify.
- Scalability – Adding new features doesn’t break existing functionality.
- Flexibility – Different implementations can be swapped easily.
- Testability – Makes unit testing easier by reducing dependencies.
- Readability – Code is cleaner and easier to understand.
Conclusion
SOLID principles help developers create robust, flexible, and maintainable software. By following these principles, software can be easily extended, tested, and managed while reducing complexity and dependency issues.